We'll walk through the steps to authenticate against the API and perform a redemption to grab a reward code, using the ubiquitous curl
.
Prerequisites
Make sure you have in hand your API Key and API Secret for the sandbox environment. For this example:
- API Key: ask a Sandbox API Key to your account manager
- API Secret: ask a Sandbox Secret Key to your account manager
Your account manager will also send you a Promotion ID representing your reward delivery campaign. For this example:
- Promotion ID: 790
1. First, let's check who we are
We'll use the API-Key based authentication and put the credentials directly in the headers as we hit the Get the current Account route.
curl 'https://api-sandbox.ifeelgoods.com/fulfillment/v2/accounts/me' \
-H 'Accept: application/json; pretty=true' \
-H 'API-Key: 2e7444c93f0d4e****************' \
-H 'API-Secret: 7f62b4c0cde*************************'
And we get a response similar to this one :}
{
"data": {
"id": 101811,
"login": null,
"name": "API Developers",
"email": "[email protected]",
"phone_number": "",
"roles": [
"fulfillment_api"
],
"locale": "en",
"timezone": "America/Los_Angeles",
"access_type": "service"
}
}
NB: We use the pretty format for readability purposes but please don't use it in production !
2. Now let's retrieve the list of rewards we are able to deliver
Your account manager has configured a selection of reward for you, let's check the details of your promotion with the Get a Promotion route.
curl 'https://api-sandbox.ifeelgoods.com/fulfillment/v2/promotions/790' \
-H 'Accept: application/json; pretty=true' \
-H 'API-Key: 2e7444c93f0d4e****************' \
-H 'API-Secret: 7f62b4c0cde*************************'
In this response, we can find the list of the rewards.
{
"data": {
"id": 790,
"ui_id": null,
"locale": "en",
"name": "Demo Campaign",
"start_datetime": "2017-04-24T07:00:00Z",
"end_datetime": null,
"client": {
"id": 303,
"name": "API Developers"
},
"status": "enabled",
"state": "ready",
"allow_pdf": true, // whether your promotion allows PDF or not.
// If true, reward_codes will include a link to the PDF
"rewards": [
{
"sku": "SBU-STT-5USD-US",
"name": "$5 Starbucks® eGift Card",
"variable_value": false, // true, if it's a "open value" reward
"state": "ready",
"status": "enabled"
},
{
"sku": "APP-ITC-5USD-US",
"name": "$5 iTunes® Code",
"variable_value": false,
"state": "no_allocation", // reward not yet configured for your account, but it can still be redeemed (ask your account manager)
"status": "enabled"
},
{
"sku": "AMZ-AMC-5USD-US",
"name": "$5 Amazon.com Gift Card",
"variable_value": false,
"state": "invalid_reward", // or "invalid_reward_for_redemption_flow", product misconfigured (Ifeelgoods issue, ask your account manager)
"status": "enabled"
},
{
"sku": "GAP-GAP-001",
"name": "$10 Gap Gift Card",
"variable_value": false,
"state": "ready",
"status": "disabled" // reward has been disabled on your account and will probably no longer be sellable
},
{
"sku": "MCD-MCC-VAR",
"name": "Ma Carte Cadeau",
"variable_value": true,
"state": "ready",
"status": "paused" // reward has been paused on your account, it will probably be sellable again later
}
],
"fraud_level": "standard",
"redeem_frequency": "multiple",
"authentication_systems": [
"email"
],
"secured_order_ids": false
}
}
Availability of Rewards
The rewards available for you to use are those which meet the following criteria :
status
isenabled
andstate
isready
orno_allocation
.
status
possible values are:enabled
,paused
anddisabled
state
possible values are:ready
,no_allocation
,invalid_reward
orinvalid_reward_for_redemption_flow
More details on
status
andstate
can be find here.If your Application or Service lets users selects gifts among a catalog of rewards, we recommend you to list only the rewards that meet these criteria, so that if - for any reason - a reward is not available, it will not be listed to your customers. In this example, you should be listing:
- SBU-STT-5USD-US
- APP-ITC-5USD-US
- GAP-GAP-001
Now let's try to get one of them.
3. Let's reward ourselves for this work !
3.1 Retrieve a reward code
Select a SKU from the previous response to get ourselves a well reserved reward using the Create and Redeem route. What about a $10 Gap Gift Card ?
curl 'https://api-sandbox.ifeelgoods.com/fulfillment/v2/promotions/790/rewards/GAP-GAP-001/redemptions/actions/redeem' \
-H 'Accept: application/json; pretty=true' \
-H 'Content-Type: application/json' \
-H 'API-Key: 2e7444c93f0d4e****************' \
-H 'API-Secret: 7f62b4c0cde*************************' \
-d '{ "data": { "user": { "email": "[email protected]" }, "order_id": "ifg4dev_order_xxx" } }'
NOTE:
order_id
must be unique.The order_id is the unique identifier allowing us to track your orders.
Our redemptions are idempotent, calling the Redeem or Create and Redeem endpoint with the same parameters multiple times will always lead to the same redemption.
- In case of timeout or failure, you can call Redeem or Create and Redeem on the same order again, it will retry the same order on our side.
- In case of success, calling the Redeem or Create and Redeem endpoint with the same parameters will always return the same redemption
Which leads to this response :
{
"data": {
"id": "ZN7RS-9RNLY",
"url": "https://redemption-sandbox.ifeelgoods.com/#/ZN7RS-9RNLY",
"state": "redeemed",
"order_id": "ifg4dev_order_xxx",
"testing": false,
"activation_datetime": "2018-12-13T16:38:14Z",
"redeemed_datetime": "2018-12-13T16:38:14Z",
"updated_datetime": "2018-12-13T16:38:14Z",
"client": {
"id": 303
},
"promotion": {
"id": 790,
"status": "enabled",
"state": "ready"
},
"authentication_systems": [
"email"
],
"notification_channels": [
"email"
],
"activation_notification_channels": null,
"reward": {
"sku": "GAP-GAP-001",
"status": "enabled",
"state": "ready"
},
"value": {
"amount": 10.0,
"currency": "USD"
},
"user": {
"id": 1253
},
"reward_format": {
"usages": ["primary", "security"] // possible values: ["primary"], ["primary", "security"], ["url"], ["security_url", "security_url_code"]
"barcode_type": "c128" // possible values: none, c128, qrcode
},
"reward_codes": {
// Exhaustive list, it will change depending on the reward you used.
// Pick the ones you need based on "reward_format.usages values".
"codes": [
// single code or code + pin
{
"name": "Gift Card Number",
"value": "GAP-10USD-TEST-08", // HERE THE GIFT CARD CODE !
"format": "string",
"usage": "primary"
},
// code + pin
{
"name": "PIN",
"value": "PIN-08",
"format": "string",
"usage": "security"
},
// single link
{
"name": "Link",
"value": "https://gift-card.partner.com/testifg21",
"format": "url",
"usage": "url"
},
// link + code
{
"name": "Security Url",
"value": "https://secure-gift-card.partner.com/testifg21",
"format": "url",
"usage": "security_url"
},
// link + code
{
"name": "Security Url Code",
"value": "12345",
"format": "string",
"usage": "security_url_code"
},
// PDF (if supported)
{
"name": "Printable document",
"value": "https://app-sandbox.ifeelgoods.com/print/ecard/evdt6-ezljp?o=1&t=e76ea718029dd60e0e2d585703b36c47",
"format": "url",
"usage": "printable_document_url"
},
// barcode
{
"name": "Barcode",
"value": "GAP-10USD-TEST-08-PIN-08",
"format": "string",
"usage": "barcode"
}
],
"expiration_datetime": null
}
}
}
Success!
Get the code from the response and be free to deliver it to the beneficiary the way you decide!
3.2 Let us send a reward code to your end-users.
The Ifeelgoods Fulfillment API also enables you to deliver the reward via email or text, direclty to your end-users.
To this purpose, we will leverage the Ifeelgoods Gifting Portal, an highly customizable userflow. Let's check how you can achieve that.
Make sure your account manager has configured your promotion to notify your end-users they received a gift in recognition for their loyalty! In our example, we will send a Gap Gift Card via email.
We will pass the email of your end-users as a parameter to the Create a Redemption route. Here [email protected]
.
curl 'https://api-sandbox.ifeelgoods.com/fulfillment/v2/promotions/790/rewards/GAP-GAP-001/redemptions' \
-H 'Accept: application/json; pretty=true' \
-H 'Content-Type: application/json' \
-H 'API-Key: 2e7444c93f0d4e****************' \
-H 'API-Secret: 7f62b4c0cde*************************' \
-d '{ "data": { "user": { "email": "[email protected]" }, "order_id": "ifg4dev-viaemail" } }'
The response will be much the same as the previous one except it does not contain any reward code and the state
remains "pending"
.
{
"data": {
"id": "SE3TY-QPXNG",
"url": "https://redemption-sandbox.ifeelgoods.com/#/SE3TY-QPXNG",
"state": "pending",
"order_id": "ifg4dev-email",
"testing": false,
"activation_datetime": "2018-12-21T12:36:56Z",
"redeemed_datetime": null,
"updated_datetime": "2018-12-21T12:37:01Z",
"client": {
"id": 303
},
"promotion": {
"id": 790,
"status": "enabled",
"state": "ready"
},
"authentication_systems": [
"email"
],
"notification_channels": null,
"activation_notification_channels": null,
"reward": {
"sku": "GAP-GAP-001",
"status": "enabled",
"state": "ready"
},
"user": {
"id": 1254
}
}
}
However, an email has been sent to the email you provided, including a link to the userflow allowing the user to access this reward. You'll find below an example of customized email.
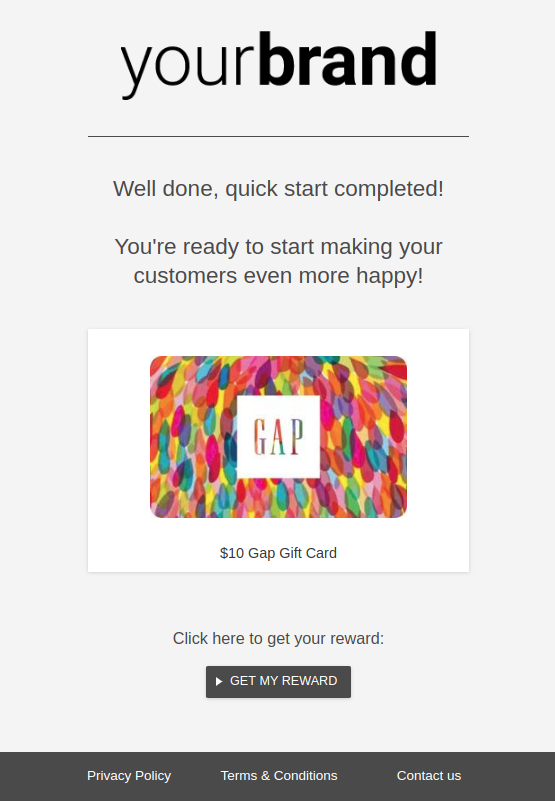
Example of customized email sent to your end-users
If you want to deliver the link on your own, you can also directly grab the link to the userflow from the response (url
attribute).
Success!
Your end-user can now enjoy his reward. This is as simple as that to make customer happy :-)